
Introduction
The Internet as we know it today is structured and streamlined extensively using Javascript. Single-page to highly sophisticated interactive websites are built from the ground up using JavaScript as a base language. Such precedence places javascript at the top of the chain, demanding prodigious approaches and solutions. Modern problems require efficient and performant solutions, and while building these is straightforward, packaging and offering them is where the true complexity lies.
The vastness of the Javascript ecosystem and the demand surge of usage brought numerous tools into the market to address the limitations. Node package manager (npm) took the spotlight and became a promising and battle-tested tool to manage and maintain Javascript packages, especially Node.js – a cross-platform environment exclusively developed with a robust runtime to create server-side javascript web applications that run and execute outside the browser.
What is npm?
The npm (node package manager) is an efficient and transcendent offering that helps overcome the complexities of packaging and distributing Javascript modules and making them available through a centralized registry to host them as installable npm dependencies.
In a nutshell, npm allows developers to build, host, and interact with shareable Javascript packages rapidly, with enhanced functionality and code reliability guarantees. The package lifecycle can be handled efficiently using three components – npm Website, Registry, and CLI.
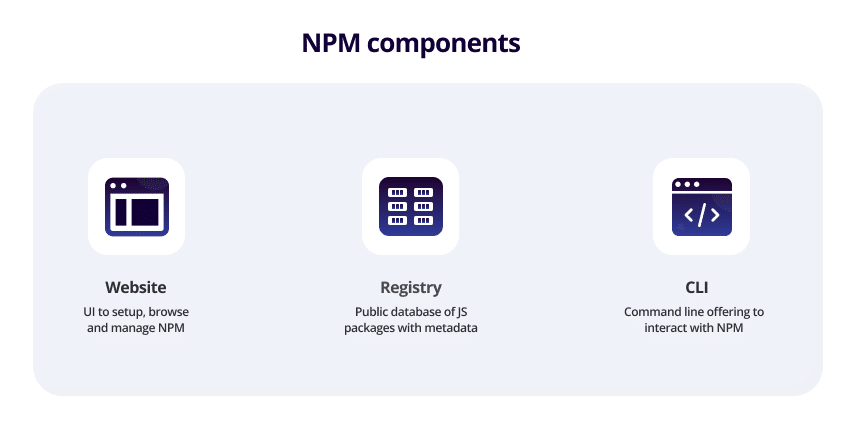
Website
The npm website acts as a centralized user interface that makes it simple to access features, packages, libraries, and administrative options within the npm ecosystem.

The website offers an extensive view of code, dependencies, version history, and package metadata with a usage guidance readme and stats with capabilities for public and private repository isolation.
Registry
The registry is the centralized hub mounted on CouchDB hosting the npm packages for querying and upserts. npm packages are generally queried via name, version, or scope. Behind the scenes, npm communicates with the registry to resolve packages by name or version to read the package information.
By default, npm points to a public registry hosting general-purpose and open-source packages with customizable configurations to leverage private registry capabilities. Registries are boundless, as one can use any compatible or custom registry (public or private) to host and offer npm packages.
CLI
The CLI (command line interface) is a tool/utility bundled with dependency handling, package management, deployment, and administrative control capabilities.

The command line tool offers programmatic access to all npm features, enabling project management to manage dependencies and automation. Developers rely excessively on npm CLI to maintain package integrity and manage dependencies.
Getting Started with npm
The rise of Node.js took the web paradigm by storm. The runtime environment has become a go-to option for developers intending to build cutting-edge modules using robust and sound principles that are battle-tested for security and performance. Understanding the npm domain and its internals holds weight if the aim is to build robust and resilient applications.
The walk-through is aimed at ensuring the reader has a thorough understanding of npm and the ecosystem and is equipped with the tools and best practices to make an impact with their innovative ideas.
Node.js Installation and Setup
Node.js is an OS-agnostic runtime environment that enables developers to tap into the capabilities of JavaScript from outside the browsers. Based on the underlying OS, there are multiple approaches for installing Node.js.
The installable binary/package can be downloaded from the Node.js official download page for setup and configuration. The installer comes in two flavors. The current flavor – with new and untested features (sometimes) and LTS (long-time support) – with stable and secure features.
To install Node.js based on OS, we can download tarball for Linux, a PKG for Mac, or MSI for Windows and install them post-decompression by following standard OS-specific package installation practices. Or install Node.js and Node package manager using the default command.
#linux sudo apt install nodejs npm #mac brew install node #windows #follow UI wizard instructions
Post execution, successful installation of the latest version of Node.js and npm can be verified using the following command.
#Node.js version validation $ node -v V20.2.0 #npm version validation $ npm -v 9.6.6
Updating npm
Node package manager is an active project, with new features released periodically. Keeping up with the npm version and migrating the old version project’s dependencies is vital to catch up with the evolving market demands and introduce new features.
npm is a command-line tool inherently pre-packed with inbuilt commands to self-update the package version and update the dependencies. The npm install command implements a predefined flow of operations to update the version.
#upgrade npm to the latest version npm install npm
Tip: An npm module npm-upgrade can be leveraged to easily update outdated dependencies with changelog inspection support and eliminate manual dependency upgrades.
Basic npm Commands
npm is the root-level entry point to work with a suite of commands for handling and administering the modules. Project setup to deployment and beyond can be achieved as npm consists of everything a developer needs to get going.
Let us explore essential npm basics and how using npm commands architects and navigates developers to build sophisticated packages:
npm Init
Modules designed and developed without structure are hard to manage and maintain. Every application needs a source of truth containing mandatory project information for reference. The npm package parses the package.json file that’s composed of necessary project metadata and configuration. For Node.js projects, the package.json file is the source of truth that gets created during the initialization of the project.
#Initialize the project npm init #Initialize the project with default values npm init -y
npm Search and Info
Searching for relevant packages and knowing about package information before installing is crucial to understanding what the package offers through the metadata. npm search and info commands help retrieve identical package metadata using package names and module descriptions in detail.

npm Install
Dependencies are the puzzle blocks responsible for shaping the final version of the project. A common pattern in Node.js applications is that they are interconnected with other publicly available promising modules in the npm registry. Most developers generally avoid the reinvention of the wheel by installing and importing public modules.
This decision introduces performant features that are tested and secure in the applications, resulting in better project structure and debugging. The command npm install will download and install packages, ensuring project dependency setup in the package.json file.
#Install a package npm install <name>
npm Start and Stop
Node.js runtime offers full-stack functionality with both client-side and server-side development. The need to control and explore the application behavior locally and on the server becomes vital. To orchestrate Node.js packages, npm equips developers with a configuration in the package.json file to modulate the behavior of application start and stop via scripts.
"scripts": { "start": "node server.js", "stop": "pkg stop server.js" }
Placing the configuration enables npm to call the parent command by parsing the JSON and referring to the Key.
#start Node.js application npm start #stop Node.js application #npm stop
Tip: npm run is a handy option to trigger custom scripts from the configuration file and npm restart is useful to restart a package when unexpected behavior is observed.
NPX
The requirement sometimes demands running one-time custom commands or using a specific version (old) of the package without installing globally to test out functionality. NPX makes it possible to run arbitrary commands from an npm package.
#run a specific version of npm package without installing it globally npx <pkg-name>@<version>
How to Initiate the First Project with npm
The primary stage of the Node.js application is to initialize it with npm for dependency management. npm init transforms the general project folder into an npm module with all the npm benefits. The resulting outcome of calling npm init is the package.json file. The package.json file is essential for managing dependencies, scripts, and metadata.
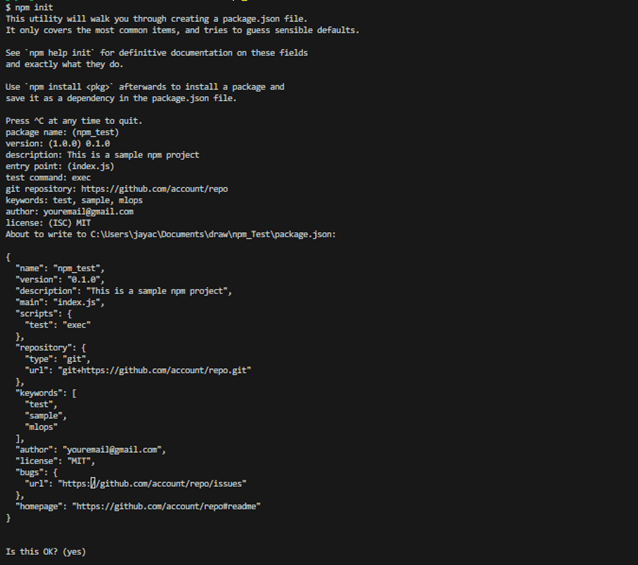
The file metadata is dependent on the keyed inputs during initialization. Although the metadata can be altered, it is important to declare the key-value pairs mindfully to avoid inconsistencies or irregularities in the application.
Package.json Metadata Properties
Understanding the metadata properties of the module is necessary to get a better grasp of npm. Initialization of npm will ensure the generation of the package.json file and the keyed values are committed to the configuration file as metadata in the current directory.
{ "name": "npm_test", "version": "0.1.0", "description": "This is a sample npm project", "main": "index.js", "scripts": { "test": "exec" }, "repository": { "type": "git", "url": "git+https://github.com/account/repo.git" }, "keywords": [ "test", "sample", "mlops" ], "author": "youremail@gmail.com", "license": "MIT", "bugs": { "url": "https://github.com/account/repo/issues" }, "homepage": "https://github.com/account/repo#readme" }
The example metadata is self-explanatory, having the project name, version, and description. The main callable js file to execute the Node.js application with the scripts that start, stop, test, and perform other administrative actions are added to scripts and main properties. The GitHub repository reference to maintain version control and log bugs are added in the repository and bugs section. The creator and usage restrictions are added via author and license properties.
Package.json Dependencies Management
All the packages installed in the application are listed under the dependency section of the package.json file. The npm manages the dependencies behind the scenes by default.
"dependencies": { "react": "^18.2.0" }
Every npm install will add a new entry to the dependency dictionary. Adding — save-dev or -D to the npm install command ensures the packages are utilized only during development.
Installing Packages
Modern web apps are dependent on various modules to operate optimally. This requirement makes the command npm install one of the most used among others. npm packages can be installed variously depending on the weightage of a respective module in local and remote execution.
Let us witness the different ways to install packages. We will try to work with the express module. A mature module to handle routes and manage servers.
Installing Packages Locally
The recommended approach is to use local package installation whenever possible to keep the modules isolated and avoid unexpected behaviors in other projects in your system directory. The npm install will install packages locally in a sub-directory named node_modules specific to the current project’s directory.
#install express module npm install express #using syntactic shortcut npm i express
Installing Global Packages
Popular modules like the express module that is useful in most applications make it a repetitive task to install packages in every project. The optimal solution is to ensure the package is installed globally and available system-wide, eliminating developer dependency.
#install express module globally npm install -g express
Tip: Packages installed globally may lead to conflicts across projects. Ensure identical version usage is carried out in all the projects.
Installing a Specific Package Version
The npm registry is indexed to enable the installation of the major version when the package name is called. Most current package versions are unstable, posing security problems and breaking existing functionality. Installing all the dependencies by dynamically passing the version is recommended.
#install 4.18.1 version of express npm install express@4.18.1
Installing from package.json
Dependency resolution is an approach that can be leveraged to predefine and install the modules and their versions if the necessary information is determined beforehand. When the list of critical dependencies is listed in package.json, the command npm install will set up the packages we want to install.
List of Installed Packages
Extracting the list of packages is necessary to understand the dependency hierarchy for debugging dependency conflicts, package version issues, and logging. Outdated packages can be pinpointed directly from npm CLI or by extracting the list to an output file.
#list npm packages npm list #list a specific package information npm list <pkg-name> #export the list to an output file npm list --json
Updating Packages
The major versions of the module that are both stable and compatible will be released periodically. To implement and experiment with new features, the module needs to be updated. The npm update command will update the package and place the reference code locally.
#update npm package npm update <pkg-name>
Uninstalling Packages
The size and dependency hierarchy of the module play a vital part in optimization and I/O. By default, npm takes care of removing unused packages through a process called pruning. But to maintain a compact and performant module, uninstalling packages from the dependency hierarchy is crucial.
#uninstall a package npm uninstall <package name>
npm performs an extra step post uninstallation by parsing all dependencies to verify if it is no longer used or dependent on the uninstalled package. If yes, the unused package will be removed as well.
Package-lock.json
Node.js being a platform and OS agnostic, module installation guarantees are a priority to ensure the applications run consistently with reproducible dependency trees, irrespective of the underlying abstraction.
Package-lock.json ensures module version and hierarchy lock to attain deterministic builds, security, and resolution.
Semantic Versioning
Promising packages bring new features and fix issues in an order. The domain-specific capabilities and restructuring of the overall package happen on the major version (X.0.0). Small and new features are introduced into root packages through minor versions (X.Y.0), and the bugs are fixed and released over patch versions (X.Y.Z). X, Y, and Z are the version pointers.
Semantic versioning is a scheme that ensures the usage of reliable and compatible changes in the application. To allow minor level changes, Caret (^) range is used — “¹.4.1”. Through Tilde (~) range — “~1.4.1” patch-level fixes are allowed, and with no range symbol, a major version will be allowed to be used.
Audit
Security management and vulnerability remediation is a key aspect of web applications. Node.js applications handle sensitive/PII information and serve it post-communication with the server. Bulletproofing the security posture is a mandate. npm audit is a brilliant offering to scrape through the project’s dependencies and flag security issues and their severity.
#Command to conduct security audit npm audit
The npm audit is a valuable command for locating and mitigating security vulnerabilities with robust monitoring capabilities.
Cache
A factor that contributes to degraded application performance is Network IO. Leveraging capabilities to eliminate repetitive and expensive operations can boost response time and functionality. The npm cache clean helps maintain a cached copy of the module for reuse.
#add npm package cache npm cache add <pkg-name>
Tip: Using npm install generally ensures a cached version is available in the default location of local packages.
Using npm Packages
Using npm packages is straightforward through mature and definitive documentation available for public reference. Hands-on and how-to solutions are at the developer’s disposal for rapid lift and shift implementations.
Getting Help
The npm is a vast domain with a thriving community. There are many means to seek help and assistance from the mature offering. npm help command is the first choice to dive into the internal workings of the npm installed packages, what they offer, and how they operate.
The npm communities and official documentation are available to find the most common problems and solutions (sometimes), and Stackoverflow can be a reliable source to seek help from npm experts and practitioners.
Tip: You can always open an issue on the npm GitHub repository and seek help from the maintainers or report bugs.
Conclusion
The tides of web development are shifting with the rise of the modern tech stack. Technological advancements are putting enterprises in a tough spot to innovate. One-size-fits-all solutions are hard to come by, but Node.js emerged as a game changer, enhancing performance and overcoming security challenges.